SQL Query & Scenarios
- benzifoo
- Dec 16, 2022
- 4 min read
SQL (Structured Query Language) is a programming language used to communicate with databases. It is a standard language for managing and manipulating data stored in relational database management systems (RDBMS).
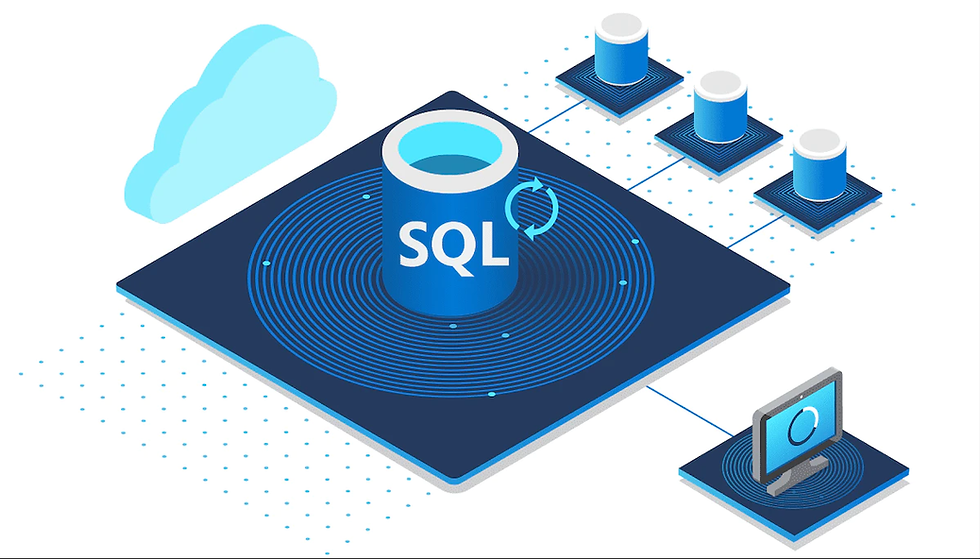
SQL is used to create, modify, and delete database objects, such as tables and indexes, and to insert, update, and delete data stored in those objects. It is also used to retrieve data from databases and perform various types of data analysis. SQL also used by many different database systems, including MySQL, Oracle, and Microsoft SQL Server. It is a versatile language that is used in many different industries, including finance, healthcare, and e-commerce.
First example of SQL query:
SELECT * FROM customers WHERE city = 'New York';
This query will retrieve all columns (indicated by the '*') from the 'customers' table where the 'city' column is equal to 'New York'.
Scenario in which this query might be used:
Imagine that you are working for a company that has a database of customer information. You have been asked to create a report that shows all of the customers who live in New York. You could use the above SQL query to retrieve this information from the database and create the report.
Second example of SQL query:
SELECT c.name, COUNT(o.id) as order_count
FROM customers c
LEFT JOIN orders o ON c.id = o.customer_id
GROUP BY c.name
HAVING COUNT(o.id) > 5
ORDER BY order_count DESC;
This query will retrieve the 'name' column from the 'customers' table (aliased as 'c') and a count of the number of orders (aliased as 'order_count') for each customer, based on the 'orders' table (aliased as 'o'). The query uses a 'LEFT JOIN' to include all customers, even if they do not have any orders. It then 'GROUP BY' the 'name' column and 'HAVING' a 'COUNT' of the 'id' column in the 'orders' table greater than 5. Finally, the results are 'ORDER BY' the 'order_count' column in descending order.
Scenario in which this query might be used:
Imagine that you are working for an online store, and you have been asked to create a report that shows the top customers by number of orders. You could use the above SQL query to retrieve this information from the database and create the report. The query would retrieve the names of all customers and a count of their orders, filter out any customers with fewer than 5 orders, and sort the results by the number of orders in descending order.
Third example of SQL query:
SELECT c.name, COUNT(o.id) as order_count, SUM(p.price * oi.quantity) as total_spent
FROM customers c
LEFT JOIN orders o ON c.id = o.customer_id
LEFT JOIN order_items oi ON o.id = oi.order_id
LEFT JOIN products p ON oi.product_id = p.id
GROUP BY c.name
HAVING COUNT(o.id) > 5
ORDER BY total_spent DESC;
This query will retrieve the `name` column from the `customers` table (aliased as `c`), a count of the number of orders (aliased as `order_count`) for each customer, and the total amount spent by each customer (aliased as `total_spent`), based on the `orders`, `order_items`, and `products` tables. The query uses `LEFT JOIN`s to include all customers, even if they do not have any orders or have only ordered items that are no longer in the `products` table. It then `GROUP BY` the `name` column and `HAVING` a `COUNT` of the `id` column in the `orders` table greater than 5. Finally, the results are `ORDER BY` the `total_spent` column in descending order.
Scenario in which this query might be used:
Imagine that you are working for an online store, and you have been asked to create a report that shows the top customers by total amount spent. You could use the above SQL query to retrieve this information from the database and create the report. The query would retrieve the names of all customers, a count of their orders, and the total amount they have spent on those orders. It would filter out any customers with fewer than 5 orders and sort the results by the total amount spent in descending order.
Fourth example of SQL query:
SELECT c.name, COUNT(o.id) as order_count, SUM(p.price * oi.quantity) as total_spent,
AVG(EXTRACT(YEAR FROM o.order_date) - c.year_of_birth) as average_age,
SUM(CASE WHEN o.order_date BETWEEN '2022-01-01' AND '2022-12-31' THEN p.price * oi.quantity ELSE 0 END) as total_spent_2022
FROM customers c
LEFT JOIN orders o ON c.id = o.customer_id
LEFT JOIN order_items oi ON o.id = oi.order_id
LEFT JOIN products p ON oi.product_id = p.id
GROUP BY c.name
HAVING COUNT(o.id) > 5
ORDER BY total_spent DESC;
This query will retrieve the `name` column from the `customers` table (aliased as `c`), a count of the number of orders (aliased as `order_count`) for each customer, the total amount spent by each customer (aliased as `total_spent`), the average age of each customer based on their year of birth and the years in which they placed orders (aliased as `average_age`), and the total amount spent by each customer in the year 2022 (aliased as `total_spent_2022`), based on the `orders`, `order_items`, and `products` tables. The query uses `LEFT JOIN`s to include all customers, even if they do not have any orders or have only ordered items that are no longer in the `products` table. It then `GROUP BY` the `name` column and `HAVING` a `COUNT` of the `id` column in the `orders` table greater than 5. The `CASE` statement is used to calculate the `total_spent_2022` column, which is the sum of the prices of all items ordered in the year 2022. Finally, the results are `ORDER BY` the `total_spent` column in descending order.
Scenario in which this query might be used:
Imagine that you are working for an online store, and you have been asked to create a report that shows the top customers by total amount spent, including their average age and the total amount they spent in the year 2022. You could use the above SQL query to retrieve this information from the database and create the report. The query would retrieve the names of all customers, a count of their orders, the total amount they have spent on those orders, the average age of each customer based on their year of birth and the years in which they placed orders, and the total amount spent by each customer in the year 2022. It would filter out any customers with fewer than 5 orders and sort the results by the total amount spent in descending order.
Comments